JControlLab
A basic graphical user interface (UI) allows to connect one or many Control Labs and send commands to the outputs. The main purpose of this UI is to offer to non-developers a easy way to run outputs with the possibility to set power or a running time.
Table of contents:
Download and Installation
Requirements
- Latest version of Java 1.8 or greater
If you use a USB-Serial adapter, the application may not work depending the hardware. JControlLab has been tested with success with Prolific compatible adapters.
Download
Executable graphical interface
Download and unzip the following archive and run "jcontrollab-0.0.1.jar".
jcontrollab-0.0.1.zip (4.6 Mo)
For development
Download the above archive and add jars to your classpath, or use the following Maven artifact:
<dependency>
<groupId>org.dajlab</groupId>
<artifactId>jcontrollab</artifactId>
<version>0.0.1</version>
</dependency>
Graphical user interface manual
The interface is available in french and english. For others languages, see this chapter.
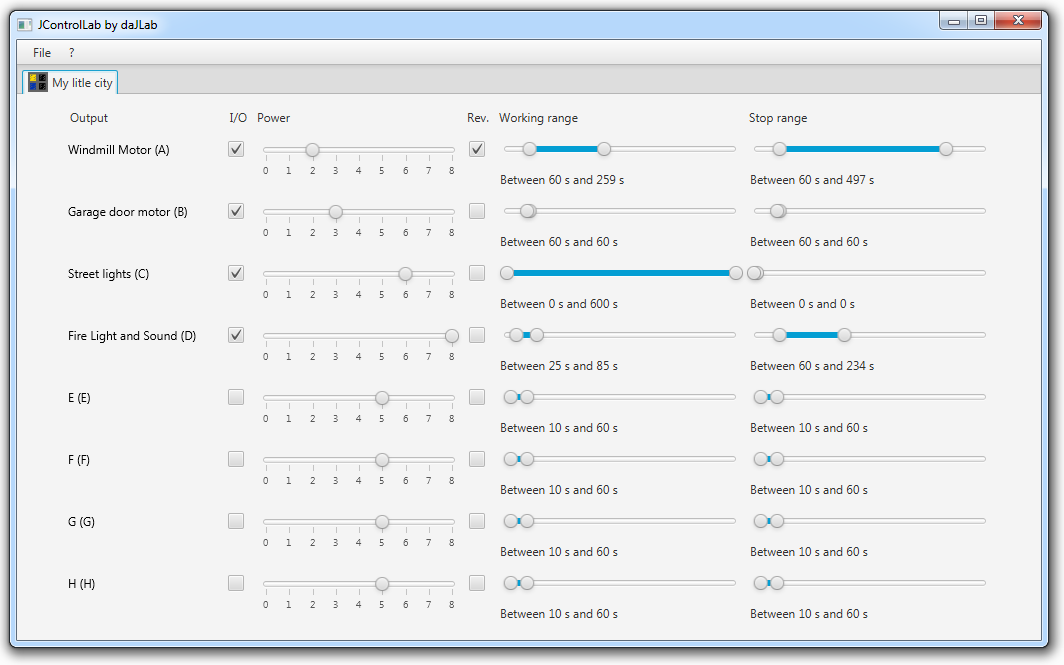
Control Lab Interfaces must be connected before the launch of the application. A tab is created for each detected devices.
For each output, you may:
- Give a label (more explicite than A, B, C...)
- Activate or not the ouput (I/O checkbox)
- Set the power
- Reverse the output
- Define the working range and stop range. A random value will be picked into the range. To run non stop an output, set the stop range to (0-0).
If you have many devices, it may be useful to give them a name. Simply double-click on the icon of a tab and change the name in the dialog box.
You can save and load the settings (see File menu). Currently, the loading system is quite basic and try to connect to the same serial port. If you change the port, the system will not be able to match the settings. You can edit the settings file with a text editor and change the serial port with the correct one. The current port is displayed in a tooltip for each tab title.
API guide
Caution: API may change with time with the inclusion of new features.
Look at org.dajlab.jcontrollab.example to see examples.
Get ControlLab instance(s)
ControlLab API is quite simple. Create an instance of ControlLabManager, call the method connect(), and then get the connected devices with getControlLabs(). Don't forget to disconnect the devices with the disconnect() method before exit.
// Create the manager
ControlLabManager manager = new ControlLabManager();
// Detect and connect the devices
manager.connect();
// Get the devices
List< ControlLabInterface> controllabs = manager.getControlLabs();
if (CollectionUtils.isNotEmpty(controllabs)) {
ControlLabInterface controlLab = controllabs.get(0);
...
}
manager.disconnect();
The outputs
Get an ouput instance from the ControlLab and send orders to it. See the Javadoc for the different controls defined by OutputInterface.
Output output = controlLab.getOuput(OutputPortEnum.A);
output.setPower(8);
output.forwardAndRun();
The inputs
JControlLab supports the 4 kind of available sensors : touch, temperature, rotation, and light sensors. Get instances from the ControlLab and add SensorListener to detect event. See the Javadoc for the different values available for each sensor.
LightSensor lightSensor = controlLab.getInput(LightSensor.class, InputPortEnum.I5);
lightSensor.addSensorListener(new SensorListener() {
@Override
public void valueChanged(SensorEvent anEvent) {
System.out.println("Light = " + ((LightSensor) anEvent.getSource()).getLight());
}
});
RotationSensor rotationSensor = controlLab.getInput(RotationSensor.class, InputPortEnum.I8);
rotationSensor.addSensorListener(new SensorListener() {
@Override
public void valueChanged(SensorEvent anEvent) {
RotationSensor rs = (RotationSensor) anEvent.getSource();
System.out.format("Rotation : raw value = %s ; %s ; angle = %2d\n", rs.getValue(),
rs.getDirection(), rs.getAngle());
}
});
TouchSensor touchSensor = controlLab.getInput(TouchSensor.class, InputPortEnum.I1);
touchSensor.addSensorListener(new SensorListener() {
@Override
public void valueChanged(SensorEvent anEvent) {
TouchSensor ts = (TouchSensor) anEvent.getSource();
if (ts.isPressed()) {
System.out.format("Touch : raw value = %s ; Sensor is pressed.\n", anEvent.getNewValue());
}
if (ts.isReleased()) {
System.out.format("Touch : raw value = %s ; Sensor is released.\n", anEvent.getNewValue());
}
}
});
TemperatureSensor tempSensor = controlLab.getInput(TemperatureSensor.class, InputPortEnum.I2);
tempSensor.addSensorListener(new SensorListener() {
@Override
public void valueChanged(SensorEvent anEvent) {
TemperatureSensor source = (TemperatureSensor) anEvent.getSource();
System.out.format("Temperature : raw value = %s <=> %.2f °C <=> %.2f °F\n",
anEvent.getNewValue(), source.getDegreesCelsius(), source.getDegreesFahrenheit());
}
});
Credits
The communication protocol with the ControlLabInterface has been reverse engineered by Andrew Carol and Anders Isaksson. Credits go to Timo Dinnesen for the Java part: