JToyPad
It provides a basic graphical user interface (UI) to connect one or many toypads, to send commands to the pads and to read tags. The main purpose of this UI is to offer to non-developers an easy way to use lights from the pads.
Table of contents:
Download and Installation
Warning: only the versions for PlayStation 3/4 and WiiU are supported. XBox versions are not supported, nobody found how to communicate with the XBox versions.
Requirements
- Latest version of Java 1.8 or greater
Driver installation
In order to use the toypad, you need to setup USB drivers.
- For Windows users (7 and more)
-
- Plug your toypad.
- Download Zadig and run it
- Select "LEGO Reader V2.10" in the devices list (if necessary, use the Options>List All Devices menu)
- Select libusbK in the list at right of the green arrow and press the install button.
- For Linux users
- Install
libusb
andlibusb1
if not already installed
- Install
Download
Executable graphical interface
Download and unzip the following archive and run "jtoypad-0.0.2.jar".
jtoypad-0.0.2.zip (3.6 Mo)
For development
Download the above archive and add jars to your classpath, or use the following Maven artifact:
<dependency>
<groupId>org.dajlab</groupId>
<artifactId>jtoypad</artifactId>
<version>0.0.2</version>
</dependency>
Graphical user interface manual
The interface is available in french and english. For others languages, see this chapter.
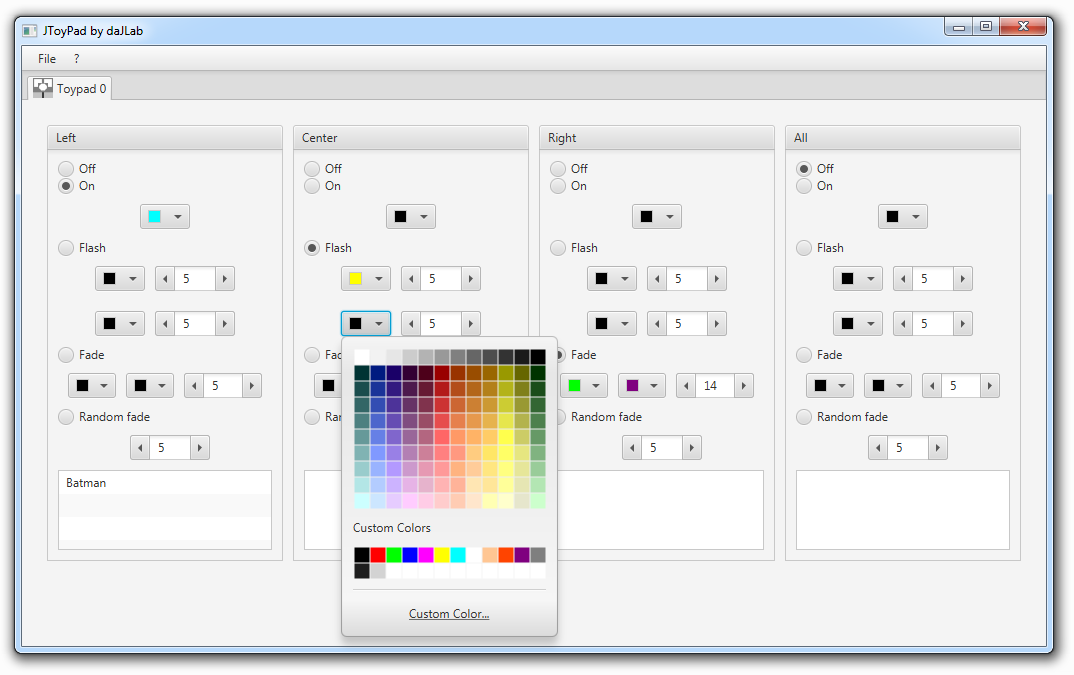
Toypads must be connected before the launch of the application. A tab is created for each detected toypad.
Commands are explicit. For a better result, select the colors among the custom colors already proposed. The list at the bottom of each pad shows the character's or vehicle's name if a LEGO Dimensions tag is present.
If you have many toypads, it may be useful to give them a name. Simply double-click on the icon of a tab and change the name in the dialog box.
You can save and load the settings (see File menu). Currently, the loading system is quite basic and try to connect to the same USB addresses. If you change the port, the system will not be able to match the settings. You can edit the settings file with a text editor and change the usb address with the correct one. The current usb address is displayed in a tooltip for each tab title.
API guide
Caution: API may change with time with the inclusion of new features.
Look at org.dajlab.jtoypad.example.SimpleExample to see a full runnable example.
Get ToyPad instance(s)
JToyPad API is quite simple. Create an instance of ToyPadManager, call the method connect(), and then get the connected toypads with getToyPads(). Don't forget to disconnect the toypads with the disconnect() method before exit.
// Create the toypad manager
ToyPadManager toypadManager = new ToyPadManager();
// Connect the toypads.
toypadManager.connect();
if (CollectionUtils.isNotEmpty(toypadManager.getToyPads())) {
// get the first one
ToyPad toypad = toypadManager.getToyPads().get(0);
...
}
// disconnect the toypads
toypadManager.disconnect();
Commands
The toypad hardware accepts predefined commands to turn lights on, fade ligths or flash lights.
Turn the three pads off. Useful when you exit the application.turnOffPads()
Switch a pad to a color.switchPad(final PadEnum pad, final Color color)
Switch all pads.switchPads(final Color colorPadLeft, final Color colorPadCenter, final Color colorPadRight)
Flash a pad. FlashColor defines the properties of the flash.flashPad(final PadEnum pad, final FlashColor flashColor)
Flash all pads.flashPads(final FlashColor flashColorLeft, final FlashColor flashColorCenter, final FlashColor flashColorRight)
Create fade for one pad. FadeColor describes the properties of the fade.fadePad(final PadEnum pad, final FadeColor fadeColor)
Fade a pad from a color to another color during the given time.fadePad(final PadEnum pad, final Color fromColor, final Color toColor, final int time)
Fade between random colors (Colors are selected by the hardware).fadePadRandom(final PadEnum pad, final int pulseTime, final int pulseCount)
Fade all pads.fadePads(final FadeColor fadeColorLeft, final FadeColor fadeColorCenter, final FadeColor fadeColorRight)
Example
toypad.flashPad(PadEnum.LEFT, new FlashColor(Color.RED, 10, Color.BLUE, 5, FlashColor.FLASH_FOR_EVER));
Read tags
To read LEGO Dimensions tags, just add a TagListener to the toypad.toypad.addTagListener(new TagListener() {
@Override
public void newTagEvent(TagEvent event) {
if (ActionEnum.ADDED == event.getAction()) {
System.out.println(event.getTag().getName());
event.getSource().switchPad(event.getPad(), Color.ORANGE);
}
}
});
Credits
The communication protocol with the toypad has been reverse engineered by many people. Credits go to:
- lego_dimensions_protocol (Python) from woodenphone. The first I started with.
- node-ld (Nodejs) from ags131. The most complete implementation with tags writing features.
- legopad_hid (C) from Lefinnois. The most well documented in my opinion.